Bitbucket API Helper Functions
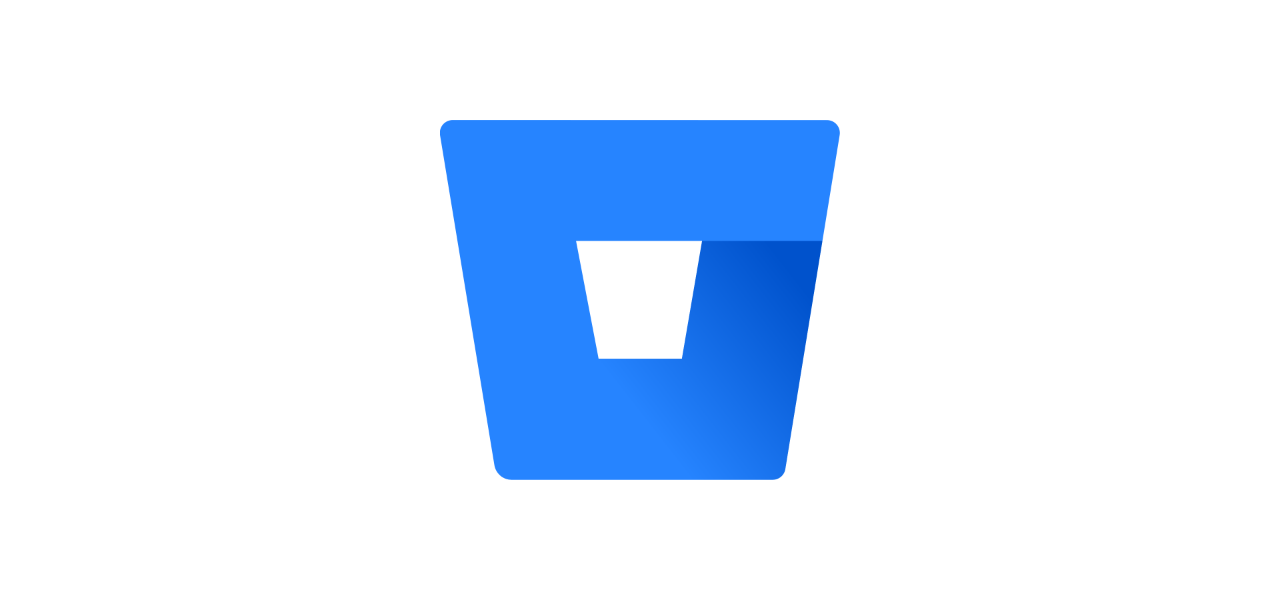
Get all pages
Normally, Bitbucket list queries return a JSON. They are structured so all the data is under a values
key. You get a bunch of other data, among which a flag isLastPage
that tells you if the page you retrieved is the last one.
I wrote below a function that iterates through pages and gets a list of results. It only depends on the the requests
python package.
def get_all_pages(base_url:str):
result = []
count = 0
limit = 50
last_page = False
prefix = "&" if base_url.find("?") > 0 else "/?"
print(f">>> {base_url}")
while not last_page:
url = base_url + f"{prefix}start={count}&limit={limit}"
response = requests.get(url, headers=HEADERS)
if response.status_code != 200:
print(f"Failed to get branches: {response.text}")
return None
repos_info = response.json()
last_page = repos_info['isLastPage']
count += limit
result += repos_info['values']
return result
Get all children
Some API requests have a different structure, where there's a path
component and list of children
:
{
"path":{
"components":["src","lib","shared","services"],
"parent":"src/lib/shared",
"name":"services",
"toString":"src/lib/shared/services"
},
"revision":"refs/heads/master",
"children":{
"size":2,
"limit":500,
"isLastPage":true,
"values":[{
"path":{
"components":["dummy.service.ts"],
"parent":"",
"name":"dummy.service.ts",
"extension":"ts",
"toString":"dummy.service.ts"},
"contentId":"content_id_for_this_file",
"type":"FILE","size":1814
},{
"path":{
"components":["search.service.ts"],
"parent":"",
"name":"search.service.ts",
"extension":"ts",
"toString":"search.service.ts"
},
"contentId":"content_id_for_this_file",
"type":"FILE",
"size":2443
}],
"start":0
}
}
In this case, the retrieval function is slightly different from the normal list of items:
def get_all_children(base_url):
result = []
count = 0
limit = 50
last_page = False
prefix = "&" if base_url.find("?") > 0 else "/?"
# print(f">>> {base_url}")
while not last_page:
url = base_url + f"{prefix}start={count}&limit={limit}"
response = requests.get(url, headers=HEADERS)
if response.status_code != 200:
print(f"Failed to get branches: {response.text}")
return None
# This should not fail
repos_info = response.json().get('children', {})
last_page = repos_info['isLastPage']
count += limit
result += repos_info['values']
return result
All these functions we'll use later to retrieve items from Bitbucket.
HTH,
PS: This is part of the RAG with Continue.dev series.