Get most recent modification date for a folder in python
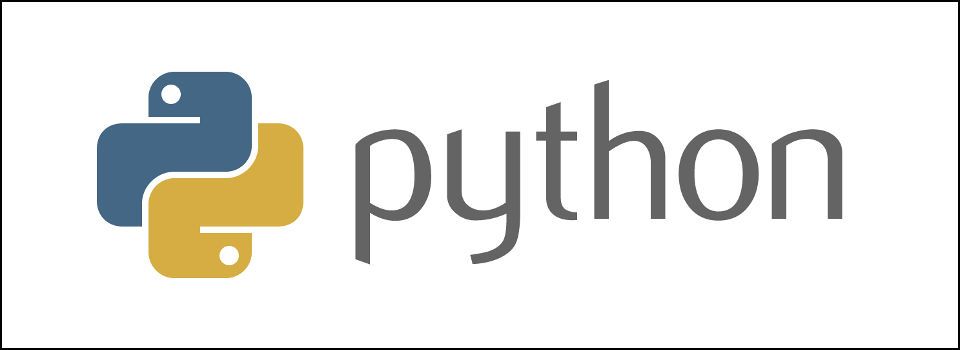
Finding out when a directory was most recently modified should be relatively easy; just perform a dir or a ls -l and you should have it. Sometimes you actually do, but not always (I remember my assembly days when I would write cool programs to manipulate the FAT information on a disk).
The most reliable way is, of course, to scan every file. To do that, I've got a simple program in two steps:
- Get the list of directories from a given path (not really related to the problem in question, but useful nonetheless and
- Scanning the folders and collecting the most recent change.
The first part is quite simple:
from os import listdir
from os.path import isdir, join
onlydirs = [ join(mypath,f) for f in listdir(mypath) if isdir(join(mypath,f)) ]
The second part uses the glorious os.walk()
method:
def get_most_recent(path):
date = os.path.getmtime(path)
file_p = None
for root, subFolders, files in os.walk(path):
for f in files:
pp = os.path.join(root,f)
d = max(os.path.getmtime(pp), os.path.getctime(pp))
if d > date:
file_p = pp
date = d
ts = time.gmtime(date)
ts = "%02d/%04d" % (ts.tm_mon, ts.tm_year)
print "%s, %s" % (ts, path)
... So that's my take on how to get most recent modification date.
It was designed as a procedure, printing the output (i.e. the date of the most recent change). One can change it to return whatever.
Note: This is not really optimised, so it's easy to figure out how it works and tweak to get whatever information you think is right.