Proper python versioning
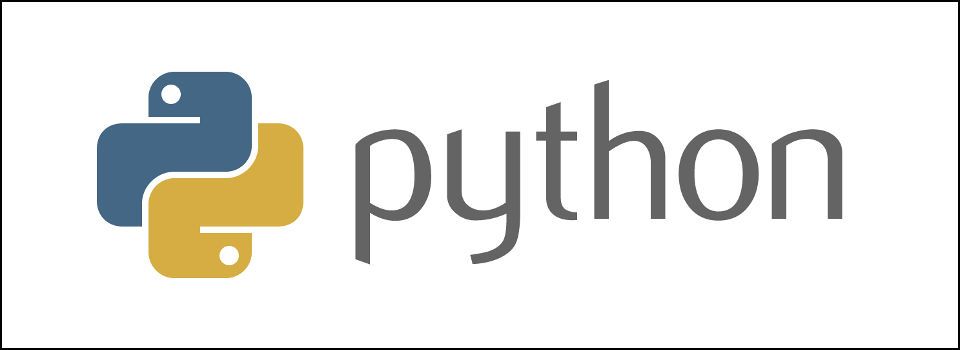
The past few days I was performing an initial deployment on a new cloud provider. During my initial runs, I have discovered a couple of snags in my applications (migration issues etc.) I've noticed that performing repetitive builds of my apps would yield the same numbers and there was no uniform way of numbering them (one had version in __init__.py
, one had it in setup.py
...).
So, I looked for an uniform way of defining versions and I found a PEP 386, doing exactly that and an implementation via __init__.py
.
My __init__.py
looks like this now:
"""
Application module
"""
from __future__ import unicode_literals
# following PEP 386: N.N[.N]+[{a|b|c|rc}N[.N]+][.postN][.devN]
VERSION = (2, 2, 0)
PREREL = ('a', 1)
POST = 0
DEV = 0
def get_version():
version = '.'.join(map(str, VERSION))
if PREREL:
version += PREREL[0] + '.'.join(map(str, PREREL[1:]))
if POST:
version += ".post" + str(POST)
if DEV:
version += ".dev" + str(DEV)
return version
__version__ = get_version()
and the setup.py
would contain something similar to:
# Code structure is
# +- setup.py
# +- src/
# +- application_module/
# +- __init__.py
# +- models.py
# ...
sys.path.append('src')
application_module = __import__('application_module')
setup(
name="application_module",
version=application_module.__version__,
# ...
)
So here's my solution to python versioning.